In this tutorial, we’ll discuss the fundamentals of multidimensional arrays and how to use them in C++ to store, access, and manipulate data.
C++ Multi-Dimensional Arrays
A multidimensional array is an array that contains more than one index or subscript. It is an “array of arrays” with many dimensions, such as three, four, or more. This type of array allows for more complex data structures and can store more information than a single-dimensional array.
Understanding The Concept of Two Dimensional Arrays
A two-dimensional array, also known as a bi-dimensional array, is a multi-dimensional array used to store tabular data. It consists of rows and columns of elements, each of which has the same data type. It can be represented by two indices, i.e., row and column index.
For example,
int X[3][6];
In the above example, the X
can hold 18 elements, and to find that, simply multiply the dimensions; in this case, 3 x 6 = 18
.
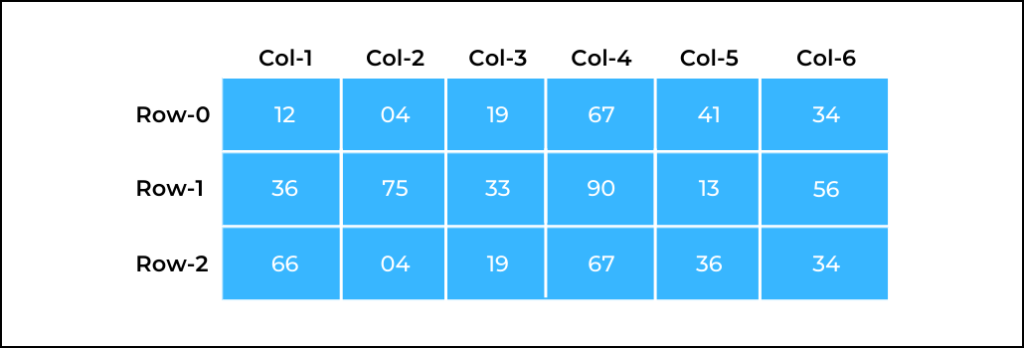
A two-dimensional array is an arrangement of data arranged in rows and columns. It can be likened to a mathematical matrix or a business table, as it can store and display information in an organized manner.
Declaration & Initialization Of Two-Dimensional Array
To use a two-dimensional array in a program, it must first be declared and initialized. This involves specifying the type of data the array will store, as well as declaring the size of each dimension and assigning values to the array’s elements. Next, you’ll learn how to Declare and Initialize a Two-Dimensional Array.
Defining/Declaring a Two-Dimensional Array
The general syntax for the declaration of two-dimensional arrays is given below:
Data_Type Array_Name [Row_Index] [Column Index];
In the above syntax, the first field in the syntax is the Data_Type. This type of data (such as int, float, char, bool, etc.) will be stored in the array. The next item is, Array_Name
which is the name of the array that will be used to refer to the array. The two final fields in the syntax are the Row_Index
and Column_Index
. These indicate the size of the array, with the Row_Index
referring to the number of rows and the Column_Index referring to the number of columns. The total size of the array will be the product of the Row_Index
and Column_Index
.
For example,
An Integer-type array, InArr, consisting of two rows and two columns, can be defined using the following statement.
int InArr [2][2];
So, this is how we can define/declare a two-dimensional array in C++. Now, let’s move to the other part of this tutorial, where we will learn about the initialization of a two-dimensional array.
Initialization of Two-Dimensional Array
To create a two-dimensional array, it is best to use a nested set of brackets, where each set of numbers inside the brackets represents a row.
//Initialization of 2D Array
int Arr[3][5] = { {2, 4, 6, 8, 10}, {8, 7, 6, 5, 4}, {5, 8, 3, 7, 2} };
We can also write the same in the following way:
//Initialization of 2D Array
int Arr[3][5] =
{
{2, 4, 6, 8, 10}, //Row 0
{8, 7, 6, 5, 4}, //Row 1
{5, 8, 3, 7, 2} //Row 2
};
This array has 3 rows and 5 columns, so we have three rows of elements with five elements each.
We can set all elements in a two-dimensional array to 0 by initializing it with {0}
.
For example,
int Arr [3][5] = {0};
Understanding The Concept Of a Three-Dimensional Array
A three-dimensional array is a data structure that stores information in a three-dimensional space. 3D arrays often represent three-dimensional objects such as cubes and other 3D shapes. They are also helpful in storing data in a tabular form.
Declaration & Initialization Of Two-Dimensional Array
To use a three-dimensional array in a program, it must first be declared and initialized. This involves specifying the type of data the array will store, as well as declaring the size of each dimension and assigning values to the array’s elements. Next, you’ll learn how to Declare and Initialize a Three-Dimensional Array.
Defining/Declaring a Three-Dimensional Array
The general syntax for the declaration of two-dimensional arrays is given below:
datatype arrayName[size1][size2][size3];
1. Datatype: This is the type of data that the array will store. This can be a primitive type (e.g., int, float, char) or a custom data type (e.g., struct).
2. ArrayName: This is the name of the array.
3. size1, size2, size3: These are the array sizes in each dimension.
Initialization Of Two-Dimensional Array
To initialize a Three-Dimensional array, the following syntax would be used:
arrayName[size1][size2][size3] = {value1, value2, ….};
For example,
Example: int cube[3][3][3] = { { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} }, { {10, 11, 12}, {13, 14, 15}, {16, 17, 18} }, { {19, 20, 21}, {22, 23, 24}, {25, 26, 27} } };
The example is an array of 3x3x3
(3 rows, 3 columns, and 3 layers) containing numbers 1 to 27. The numbers are organized in 3 layers, each containing 3 rows and 3 columns. Each layer contains the numbers 1 to 9, 10 to 18, and 19 to 27, respectively.
Accessing and Writing at an Index in 2D & 3D Array
Manipulating data in two-dimensional arrays requires accessing and updating the elements in the array. This is done similarly to a one-dimensional array by writing at an index of the array when declaring it or accessing and writing at an index of the array when working with it.
Accessing Two-Dimensional Arrays
To access a two-dimensional array, two indices are needed – one for the row and one for the column. For example, if we have an array Arr[3][5]
and want to access the element in the second row and third column, it can be accessed by writing Arr[1][2]
.
When dealing with a two-dimensional array, it is more efficient to use nested loops to access individual elements. The outer loop would iterate through the rows, while the inner loop would iterate through the columns. This method makes accessing specific elements in the array much quicker and easier.
When working with two-dimensional arrays, it is usually best to use a nested loop structure. The outer loop should iterate over the rows, and the inner loop should iterate over the columns. This allows us to access each element of the array systematically.
2D Array Example: Accessing The Elements Of The Array
#include <iostream> using namespace std; int main() { int Arr[3][5] = { {1, 2, 3, 4, 5}, {6, 7, 8, 9, 10}, {11, 12, 13, 14, 15} }; cout << "The elements of the array are:" << endl; for(int i = 0; i < 3; i++) { for(int j = 0; j < 5; j++) cout << Arr[i][j] << " "; cout << endl; } return 0; }
OUTPUT OF THE PROGRAM
The elements of the array are:
1 2 3 4 5
6 7 8 9 10
11 12 13 14 15
Here’s how the above code work:
Step 1: The first step is to declare the array. This is done using the following code:
int Arr[3][5] = { {1, 2, 3, 4, 5},
{6, 7, 8, 9, 10},
{11, 12, 13, 14, 15} };
Step 2: The next step is to print out the elements of the array. This is done by using a nested loop. The outer loop iterates through each row, and the inner loop iterates through each column. The following code is used to iterate through the array and print out the elements:
for(int i = 0; i < 3; i++)
{
for(int j = 0; j < 5; j++)
cout << Arr[i][j] << " ";
cout << endl;
}
Writing at an Index of Two Dimensional Array
Loop is a better way of writing the indices of a two-dimensional array. It allows you to iterate through all of the elements in the array without having to write separate statements for each element. This can make your code more efficient and less prone to errors. Additionally, using a for loop can help you avoid manually keeping track of the indices.
2D Array Example: Writing at an Index in 2D Array Using LOOP
#include <iostream> using namespace std; int main() { int row, column; // Prompt the user to enter the number of rows and columns cout << "Enter the number of rows: "; cin >> row; cout << "Enter the number of columns: "; cin >> column; // Declare a 2D array with the specified dimensions int X[row][column]; // Prompt the user to enter values for the array cout << endl << "Enter values for the array:" << endl; // Input values into the array for (int i = 0; i < row; i++) { for (int j = 0; j < column; j++) { cin >> X[i][j]; } } // Display the values of the array cout << endl << "Values of the array are:" << endl; for (int i = 0; i < row; i++) { for (int j = 0; j < column; j++) { cout << X[i][j] << "\t"; // Accessing the array } cout << endl; } return 0; }
OUTPUT OF THE PROGRAM
Enter the number of row: 2
Enter the number of column: 3
Enter Values For The Array:
4
7
2
3
1
9
Values Of The Array are:
4 7 2
3 1 9
This program prompts the user to input an array’s number of rows and columns. It then asks the user to input values for the array. The values are then stored in the array. Finally, it prints the values of the array.
Example 3: Three-Dimensional Array
#include <iostream> using namespace std; int main() { // Ask the user for the array size int x, y, z; cout << "Enter the x, y and z size of the array: "; cin >> x >> y >> z; // Declare a 3D array with the size provided by the user int myArray[x][y][z]; // Ask the user to enter each value for (int i = 0; i < x; ++i) { for (int j = 0; j < y; ++j) { for (int k = 0; k < z; ++k) { cout << "Enter the value for myArray[" << i << "][" << j << "][" << k << "]: "; cin >> myArray[i][j][k]; } } } // Displaying the values with the proper index. cout<<endl; for (int i = 0; i < x; ++i) { for (int j = 0; j < y; ++j) { for (int k = 0; k < z; ++k) { cout << "myArray[" << i << "][" << j << "][" << k << "] = " << myArray[i][j][k] << endl; } } } return 0; }
OUTPUT OF THE PROGRAM
Enter the x, y and z size of the array: 2
3
2
Enter the value for myArray[0][0][0]: 2
Enter the value for myArray[0][0][1]: 5
Enter the value for myArray[0][1][0]: 2
Enter the value for myArray[0][1][1]: 5
Enter the value for myArray[0][2][0]: 8
Enter the value for myArray[0][2][1]: 1
Enter the value for myArray[1][0][0]: 4
Enter the value for myArray[1][0][1]: 9
Enter the value for myArray[1][1][0]: 4
Enter the value for myArray[1][1][1]: 6
Enter the value for myArray[1][2][0]: 7
Enter the value for myArray[1][2][1]: 0
myArray[0][0][0] = 2
myArray[0][0][1] = 5
myArray[0][1][0] = 2
myArray[0][1][1] = 5
myArray[0][2][0] = 8
myArray[0][2][1] = 1
myArray[1][0][0] = 4
myArray[1][0][1] = 9
myArray[1][1][0] = 4
myArray[1][1][1] = 6
myArray[1][2][0] = 7
myArray[1][2][1] = 0
The above output displays the values in the 3D array with the proper indexes. The user is asked to enter the array size, which is then used to declare a 3D array. The user is then asked to enter each value in the array, which is then displayed with the proper index.